Server-side source code, Kdt750 apl guide – AML KDT750 Price Verification System User Manual
Page 28
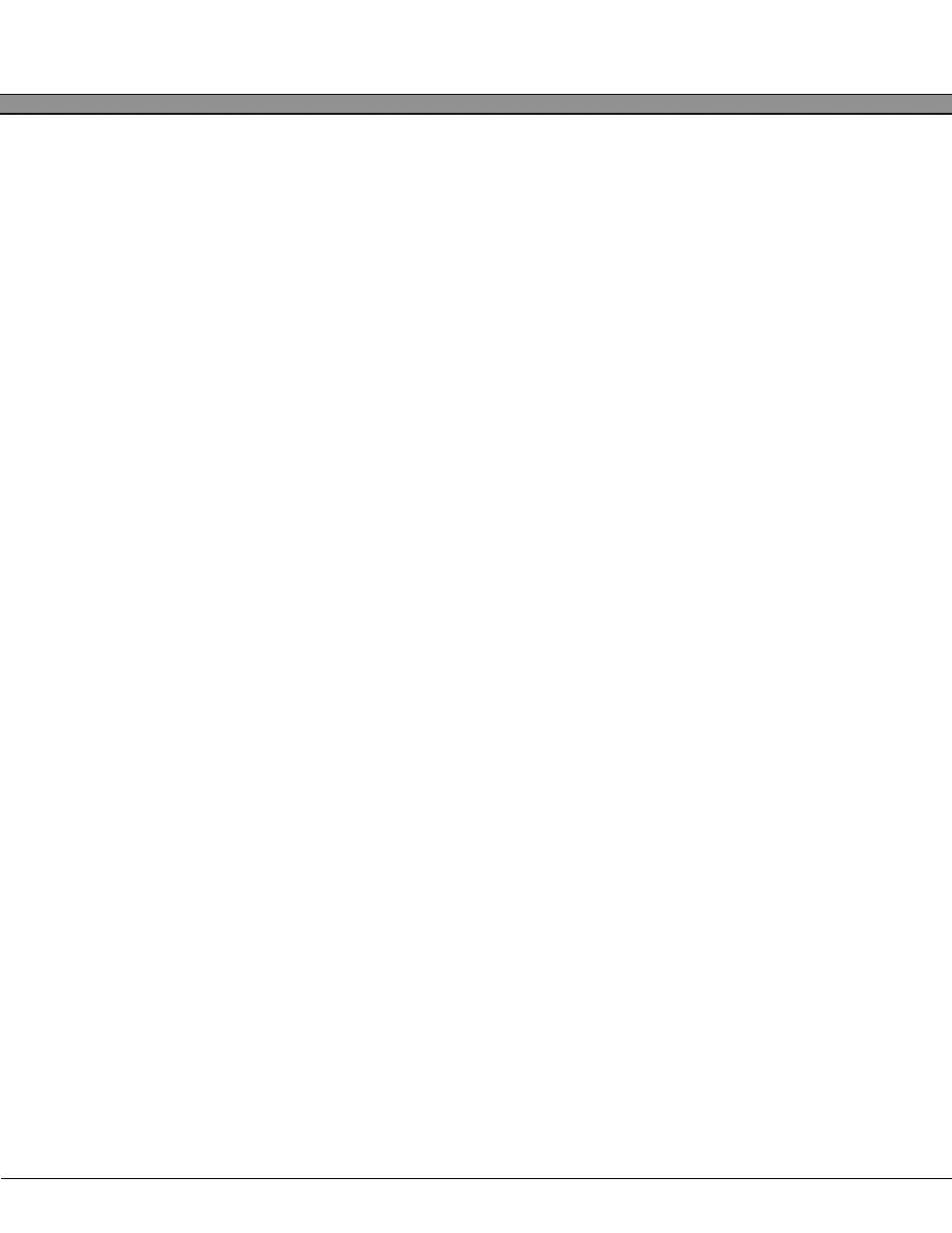
KDT750 APL Guide
4 - 6
Server-side Source Code
# Use threads and sockets
import socket
import thread
# Lookup thread function to be called when a client connects
def lookup_thread ( client_socket, address ):
# Read the request up to 512 bytes
data = client_socket.recv ( 512 )
# We don't care about which input device it came from for this example
data = data.lstrip( '\002' ) # Remove <STX>
data = data.rstrip( '\003' ) # Remove <ETX>
device, barcode = data.split ( '=' ) # Split the parts
# Do database work
prc = do_database ( “SELECT Price FROM MyDatabase WHERE Barcode IS ” barcode )
des = do_database ( “SELECT Description FROM MyDatabase WHERE Barcode IS ”
barcode )
# Send “Unknown Item” on no record found
if prc == ''
reply = '\002' “FIELD1=Unknown Item” '\000'
"@FIELD1=125,145,510,250,219,255 '\003'
else :
reply = '\002' “PRC=” prc '\000' “DES=” des '\003'
# Send the reply and disconnect the client
client_socket.send ( reply )
client_socket.close ( )
return
# Create the INET streaming socket
server_socket = socket.socket ( socket.AF_INET, socket.SOCK_STREAM )
# Bind to an unused TCP port - say 5000...
server_socket.bind ( ( "", 5000 ) )
# Allow 5 concurrent connections at a time
server_socket.listen ( 5 )
# Loop forever, handing new clients off to their own thread
while 1:
client_socket, address = server_socket.accept ( )
thread.start_new_thread ( lookup_thread, ( client_socket, address ) )