IBASE SMARC-EVK1 User Manual
Page 76
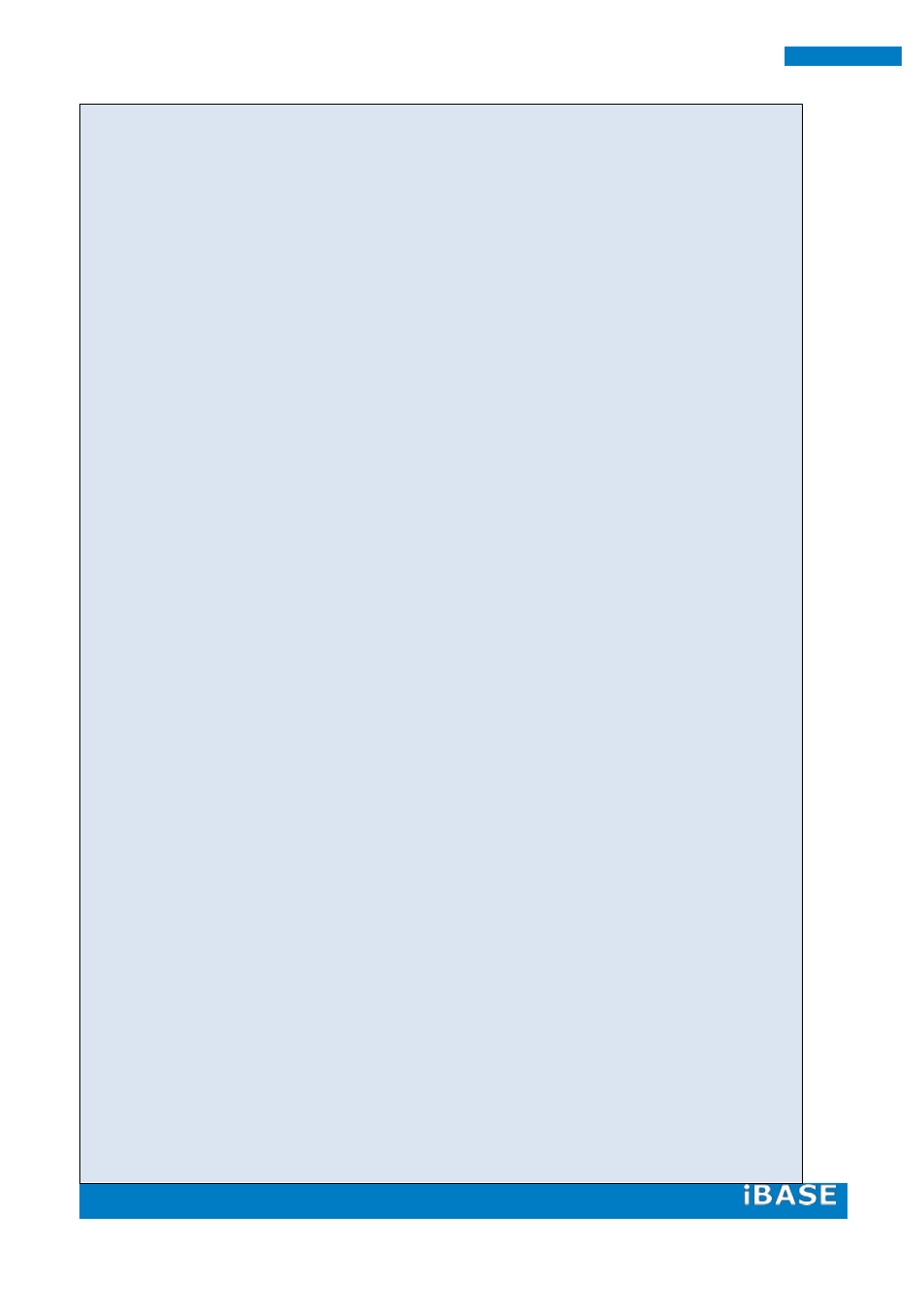
50
SMARC Evaluation Kit
#define _BSD_SOURCE 1
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/param.h> /* for NAME_MAX */
#include <sys/ioctl.h>
#include <string.h>
#include <strings.h> /* for strcasecmp() */
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <limits.h>
#include <dirent.h>
#include <fcntl.h>
#include <errno.h>
#include "i2cbusses.h"
#include <linux/i2c-dev.h>
enum adt { adt_dummy, adt_isa, adt_i2c, adt_smbus, adt_unknown };
struct adap_type {
const char *funcs;
const char* algo;
};
static struct adap_type adap_types[5] = {
{ .funcs = "dummy",
.algo = "Dummy bus", },
{ .funcs = "isa",
.algo = "ISA bus", },
{ .funcs = "i2c",
.algo = "I2C adapter", },
{ .funcs = "smbus",
.algo = "SMBus adapter", },
{ .funcs = "unknown",
.algo = "N/A", },
};
static enum adt i2c_get_funcs(int i2cbus)
{
unsigned long funcs;
int file;
char filename[20];
enum adt ret;
file = open_i2c_dev(i2cbus, filename, sizeof(filename), 1);
if (file < 0)
return adt_unknown;
if (ioctl(file, I2C_FUNCS, &funcs) < 0)
ret = adt_unknown;
else if (funcs & I2C_FUNC_I2C)
ret = adt_i2c;
else if (funcs & (I2C_FUNC_SMBUS_BYTE |
I2C_FUNC_SMBUS_BYTE_DATA |
I2C_FUNC_SMBUS_WORD_DATA))
ret = adt_smbus;
else
ret = adt_dummy;
close(file);
return ret;
}
/* Remove trailing spaces from a string
Return the new string length including the trailing NUL */
static int rtrim(char *s)
{
int i;
for (i = strlen(s) - 1; i >= 0 && (s[i] == ' ' || s[i] == '\n'); i--)