Applied Motion SV7-Q-EE User Manual
Page 19
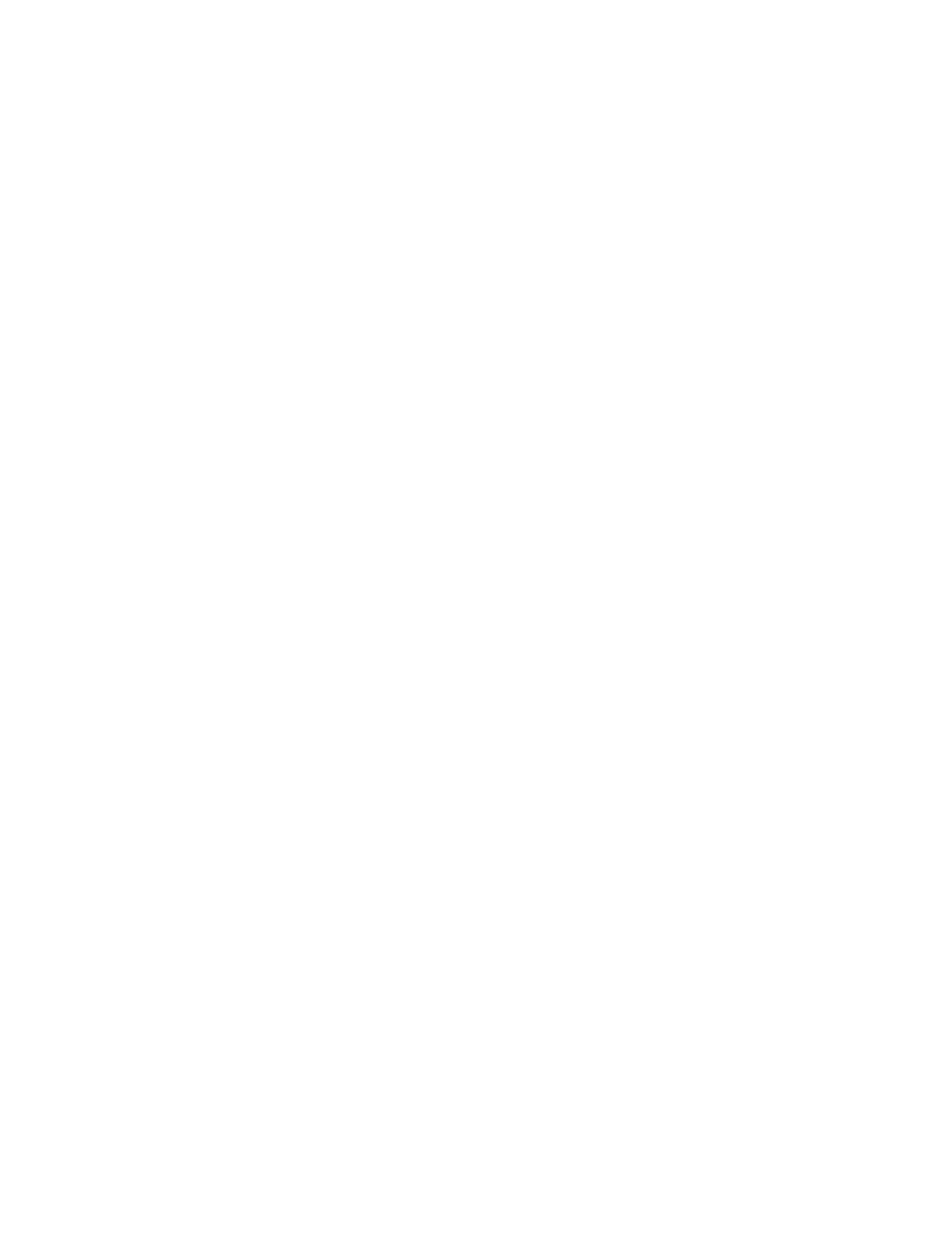
6/26/2010
920‐0032a3 eSCL Communication Reference Manual
Page 19
must specify the protocol as TCP, choose a local port number, and set the remote IP address and port number
to match the drive. In the code example below, 7776 is the port of the drive. driveIPaddress is the IP address
of the drive (“10.10.10.10” or “192.168.0.130” for example).
Winsock1.RemotePort = 7776
Winsock1.RemoteHost = driveIPaddress
Winsock1.Protocol = sckTCPProtocol
Sending “RV” command:
Dim myPacket(0 to 4) as Byte ‘ declare a byte array just large enough
myPacket(0) = 0
‘ first byte of SCL opcode
myPacket(1) = 7
‘ second byte of SCL opcode
myPacket(2) = “R”
‘ R
myPacket(3) = “V”
‘ V
myPacket(4) = vbCR
‘ carriage return
‘ after 20 seconds of inactivity, the drive will close the connection
‘ resulting in a Winsock state error
‘ check for the error and close this end of the connection
If Winsock1.State = sckError Then Winsock1.Close
‘ if necessary, open a new connection
If Winsock1.State = sckClosed Then
Winsock1.Connect
Delay 0.1
‘ 0.1 second delay
End If
If Winsock1.State = sckConnected Then
Winsock1.SendData myPacket
End If
To receive a response, you will need to place some code in the Winsock_DataArrival event. This event is
automatically declared as soon as you add a Winsock control to your form. The DataArrival event will
automatically trigger each time a packet is received. The code below extracts the SCL response from the
payload and displays it in a message box.
Private Sub Winsock1_DataArrival(ByVal bytesTotal As Long)
Dim udpData() As Byte, n As Integer
Dim hexbyte As String, packetID As Long, SCLrx As String
Winsock1.GetData udpData
' remotehost gets clobbered when packet rec'd,
‘ next line fixes it
Winsock1.RemoteHost = Winsock1.RemoteHostIP
' first 16 bits of packet are the ID (opcode)
If UBound(udpData) >= 1 Then
packetID = 256 * udpData(0) + udpData(1)
If packetID = 7 then ' SCL response
SCLrx = ""
For n = 2 To UBound(udpData)
SCLrx = SCLrx & Chr(udpData(n))
Next n
MsgBox SCLrx
End If
End If
End Sub