Applied Motion RS-232 User Manual
Page 280
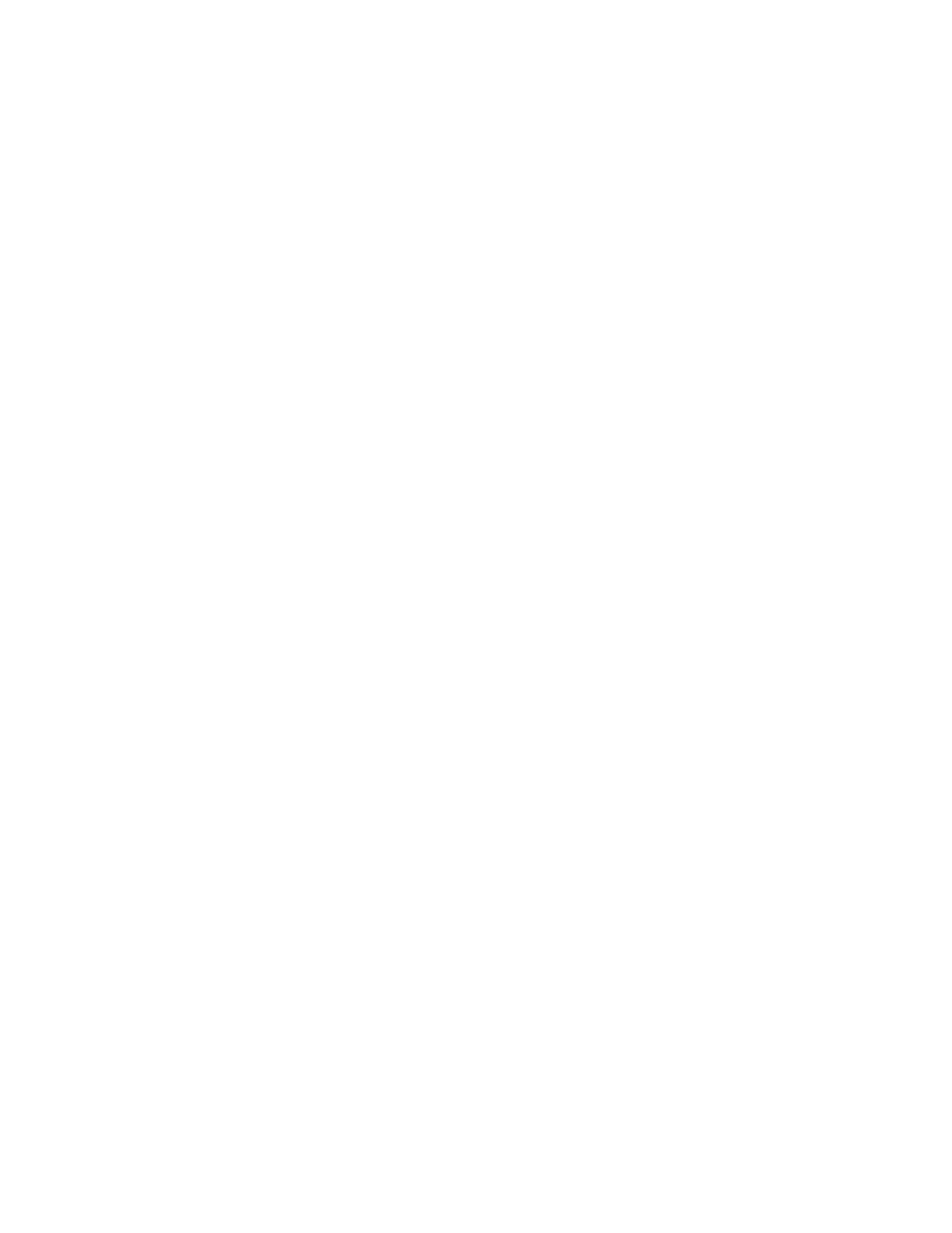
280
920-0002 Rev. I
2/2013
Host Command Reference
Creating a receive event using a call back function
First, create a function to handle incoming packets. This function must contain two local objects: a
UdpClient and an IPEndPoint. The call back function will be passed an IAsyncResult object that contains a
reference to the UDP connection. The local IPEndPoint object is passed to the UDPClient’s EndReceive property
to retrieve the packet.
public void
ReceiveCallback(
IAsyncResult
ar)
{
int
opcode;
UdpClient
u = (
UdpClient
)((
UdpState
)(ar.AsyncState)).u;
IPEndPoint
e = (
IPEndPoint
)((
UdpState
)(ar.AsyncState)).e;
Byte
[] receiveBytes = u.EndReceive(ar,
ref
e);
// get opcode
opcode = 256 * receiveBytes[0] + receiveBytes[1];
if
(opcode == 7)
// SCL response
{
string
receiveString =
Encoding
.ASCII.GetString(receiveBytes);
Byte
[] SCLstring =
new
Byte
[receiveBytes.Length - 2];
// remove opcode
System.
Array
.Copy(receiveBytes, 2, SCLstring, 0, SCLstring.
Length);
receiveString =
Encoding
.ASCII.GetString(SCLstring);
AddToHistory(receiveString);
}
else if
(opcode == 99)
// ping response
{
MessageBox
.Show(
“Ping!”
,
“eSCL Utility”
,
MessageBoxButtons
.OK,
MessageBoxIcon
.Information);
}
}
The call back function will not be called unless it is “registered” with the UdpClient object using the
BeginReceive method, as shown below. StartRecvCallback can be called from the Form Load event. It must also
be re-registered each time it is called (this is to prevent recursion), which is most easily accomplished by making a
call to StartRecvCallback each time you send a packet.
private void
StartRecvCallback()
{
UdpState
s =
new
UdpState
();
s.e = new
IPEndPoint
(
IPAddress
.Any, 0);
s.u = udpClient;
udpClient.BeginReceive(
new
AsyncCallback
(ReceiveCallback), s);
}
This example requires that you declare a class called UdpState as described below.
class
UdpState
{
public
UdpClient
u;
public
IPEndPoint
e;
}
As if this event driven technique wasn’t quirky enough, it also creates a threading error unless the following
statement in included in the form load event
// this must be so for callbacks which operate in a different thread
CheckForIllegalCrossThreadCalls =
false
;